반응형
멀티 쓰레드는 GIL로 인해 자원 손실이 존재하지만 기본적으로 I/O 대기 시간을 이용해서 실행하는 구조라고 할 수 있다.
Thread를 활용할 수 있는 방법은 threading 라이브러리를 이용할 수 있으며, 가장 기본적인 방법은 다음과 같다.
from threading import Thread
import time
def thread_task(number):
print('thread start', number)
time.sleep(int(number))
print('thread end', number)
start = time.time()
for x in range(1, 10):
thread = Thread(target = thread_task, args = [x])
thread.start()
end = time.time()
runtime = end - start
print(f'실행 시간: {runtime}')
기본 실행시간은 1초가 걸리지 않고 이후 쓰레드에서 작업을 처리하면 전체가 완료되는 시간이 10초가 걸리게 된다.
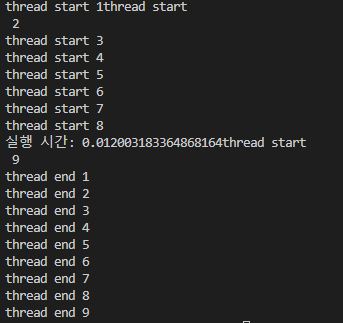
만약 스레드가 실행이 완료되는 것을 확인후 다음으로 진행하고자 한다면, join을 이용할 수 있다.
for x in range(1, 10):
thread = Thread(target = thread_task, args = [x])
thread.start()
thread.join()
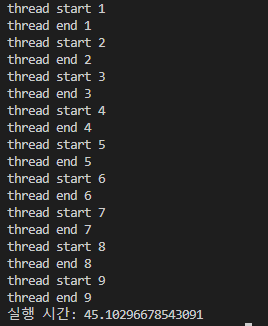
하지만 join을 이용하면 병렬로 처리한 방식과 유사하기 때문에 쓰레드를 관리할 수 있는 class를 생성해서 현재 생성된 스레드를 리스트에 넣고 빼기를 통해 전체 스레드 처리를 확인할 수 있다.
주요한 핵심은 리스트에서 스레드를 넣고 빼기 위해 유니크한 이름을 지정해 주어서 실행중인 스레드 리스트를 관리하는 방식이다.
from threading import Thread
import time
class Therad_Pool():
def __init__(self):
self.threads = [] #현재 쓰레드를 담는 리스트 생성
def insert(self, threadname):
self.threads.append(threadname)
def delete(self, threadname):
self.threads.remove(threadname)
def thread_task(number):
thread_number = f'thread{number}'
print('thread start', number)
time.sleep(number)
print('thread end', number)
thread_list.delete(thread_number) # 작업이 완료되면 현재 리스트에서 제거한다.
start = time.time()
thread_list = Therad_Pool()
for x in range(1, 10):
thread = Thread(target = thread_task, args = (x, ))
thread_number = f'thread{x}'
thread_list.insert(thread_number) # 쓰레드 이름을 통해서 실행할 때 넣는다.
thread.start()
while True:
if len(thread_list.threads) == 0: # 리스트에 스레드가 없다면 종료
break
end = time.time()
runtime = end - start
print(f'실행 시간: {runtime}')
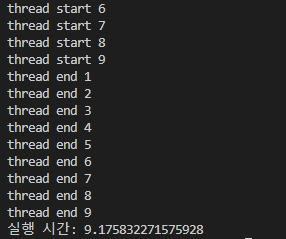
파이썬에서 쓰레드를 활용하는 방법으로 쓰레드를 생성하는 방법도 있지만, 하나의 스레드를 통해 코루틴를 활용 하는 것도 가능하다. Python 3.5 버전에 추가된 코루틴은 무엇일까?코루틴 관련해서는 아래에 정리해봤으니 관김이 있다면 살펴보면 좋을듯하다.
Python - 코루틴과 이벤트루프 이해 정리 (asecurity.dev)
Python - 코루틴과 이벤트루프 이해 정리
코루틴이란!? 코루틴은 특정 함수의 루프등을 실행하다가 일시 정지하고 재개할 수 있는 구성 요소를 말한다. 쉽게 말해 필요에 따라 일시 정지할 수 있는 함수를 의미한다,. 코루틴을 사용하여
asecurity.dev
반응형
'Python' 카테고리의 다른 글
Python - argument after * must be an iterable, not int (0) | 2024.03.25 |
---|---|
Python - 코루틴과 이벤트루프 이해 정리 (0) | 2024.03.25 |
SQLAlchemy - QueuePool limit of size 5 overflow 10 reached, connection timed out, timeout 30.00 (0) | 2024.03.25 |
Python - GIL, 멀티 쓰레드(thread) vs 멀티 프로세스(multiprocessing, subprocess) (0) | 2024.03.25 |
Python - *args와 **kwargs 이해 (0) | 2024.03.25 |