Python
Python - 스플렁크(Splunk) Query 검색
올엠
2024. 6. 8. 10:07
반응형
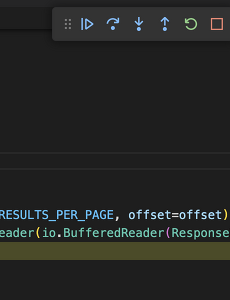
Splunk 는 외부에서 손쉽게 쿼리 검색등 다양한 작업을 할 수 있도록 지원하고 있다.
그중 쿼리 검색에 대해서 알아보도록 하자.
먼저 Splunk 라이브러리 splunk-sdk를 설치하자.
pip install splunk-sdk
이후 아래와 같은 코드를 통해 쿼리 검색이 가능하다.
import splunklib.client as client
# 스플렁크 연결
HOST = "splunk_host"
PORT = 8089
USERNAME = "account_username"
PASSWORD = "account_password"
# 검색 쿼리 설정
SEARCH_QUERY = "search index=my_index | stats count by _time"
# 스플렁크 연결
service = client.connect(
host=HOST,
port=PORT,
username=USERNAME,
password=PASSWORD
)
# 작업 실행
job = service.jobs.create(SEARCH_QUERY)
# 대기
while not job.is_done():
pass
# 결과 확인
for result in job.results():
print(result)
위와 같이 진행하여 검색 결과를 가져올 수 있다.
추가로 스플렁크는 쿼리 한번에 최대 50000건의 데이터를 가져올 수 있다.
따라서 더 많은 데이터를 가져오기 위해서는 Job 코드부분 부터 아래와 같이 수정해야 한다.
예제에서는 데이터를 표시하지는 않고 전체 카운트만 하는 코드로 작성했다.
그리고 results를 표현하기 위한 방안으로 아래와 같은 코드를 추가하여 사용하면 보다 빠르게 처리가 가능하다.
class ResponseReaderWrapper(io.RawIOBase):
def __init__(self, responseReader):
self.responseReader = responseReader
def readable(self):
return True
def close(self):
self.responseReader.close()
def read(self, n):
return self.responseReader.read(n)
def readinto(self, b):
sz = len(b)
data = self.responseReader.read(sz)
for idx, ch in enumerate(data):
b[idx] = ch
return len(data)
import splunklib.results as es_results
# 1회에 가져올 최대 데이터
RESULTS_PER_PAGE = 50000
all_results = []
total_counts = int(job['resultCount'])
if total_counts:
# 50000건 이상 데이터 가져오기
while(offset < total_counts):
serch_result = job.results(count=RESULTS_PER_PAGE, offset=offset, output_mode='json')
for result in es_results.ResultsReader(io.BufferedReader(ResponseReaderWrapper(serch_result))):
all_results.append(dict(result))
그리고 위 코드는 과거 사용하던 방식으로 최근에는 JSON을 이용해서 결과를 바로 표현할 수 있도록 지원해주고 있다.
위 코드를 아래와 같이 수정하여 JSONResultsReader 를 통해 보다 간편하게 코드를 작성할 수 있다.
total_counts = int(job['resultCount'])
if total_counts:
# 50000건 이상 데이터 가져오기
while(offset < total_counts):
serch_result = job.results(count=RESULTS_PER_PAGE, offset=offset, output_mode='json')
for result in es_results.JSONResultsReader(serch_result):
all_results.append(result)
Display search results | Documentation | Splunk Developer Program
https://dev.splunk.com/enterprise/docs/devtools/python/sdk-python/howtousesplunkpython/howtodisplaysearchpython/
dev.splunk.com
반응형